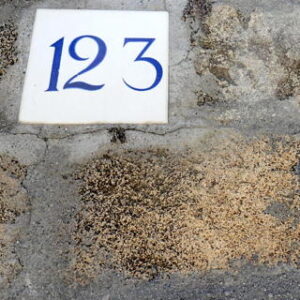
1-2-3 PHP operators
I learnt my PHP on a European keyboard, and it was always a pain to use some strange symbols. When I moved to Canada, I quickly realized that the chosen symbols were actually very easily accessible on the keyboard: it was just my layout that was less practical.
Then, I also realized how these symbols are repeatedly used to make larger ones. There is the single =
for assignation, then the double ==
for equality and then the triple ===
for identity. It is probably easier to repeat an existing symbol, than to summon a new one, which might be cumbersome to reach on the keyboard.
There is a bit of laziness in this pattern. ==
might easily mistaken with ===
(and vice versa) and the mistake might be begnin (or not), but mistaking =
and ==
is, most of the time, a bug.
Here is as selection of 1-2-3 PHP operators, along with their documentation.
1 | 2 | 3 |
---|---|---|
. | .. | … |
+ | ++ | +++ |
– | — | — |
* | ** | *** |
/ | // | /// |
> | >> | >>> |
< | << | <<< |
= | == | === |
@ | @@ | @@@ |
? | ?? | ??? |
: | :: | ::: |
! | !! | !!! |
$ | $$ | $$$ |
Dots in PHP
.
is the operator of string concatenation...
is a syntax error. It is not supported in PHP. In other language, it denotes a range between two operators....
is the ellipsis operator, to spread or collect values out or in an array
Plus in PHP
+
is the addition operator, for numbers and arrays.++
is the increment operator. It comes in two flavors, pre-increment or post increment.+++
is a syntax oddity, where there is no space between an incremented variable and its addition with another value:$b = $a+++ 1;
is$b = $a++ + 1;
Minus in PHP
-
is the substraction operator, for numbers only.--
is the decrement operator. It comes in two flavors, pre-increment or post increment.---
is a syntax oddity, where there is no space between a decremented variable and its substraction with another value:$b = $a--- 1;
is$b = $a-- - 1;
Star in PHP
*
is the multiplication operator, for numbers.**
is the power operator. It is the multiplication of multiplications, basically.***
is not supported. Math has a notion of towers of powers, where4 *** 4
would be4 ** (4 ** 4)
. That number is already beyond the integer range, so the operator is probably unuseful.
Slash in PHP
/
is the decimal division operator, for numbers.//
is a one line comment.///
is a one line comment, starting with a slash.
Greater than in PHP
>
is the greater than comparison operator.>>
is the right bitshift operator, which moves bits to the right.>>>
is not supported.
Lesser than in PHP
<
is the lesser than comparison operator.<<
is the left bitshift operator, which moves bits to the left.<<<
is the start of the Heredoc and Nowdoc sequence.
Equal in PHP
=
is the assignation operator, which gives a value to a variable.==
is the comparison operator. Types are adapted before the comparison actually happens.===
is the identity operator, which compares values and their types.
Question in PHP
?
is half the ternary operator, along with the colon.??
is the null coalesce operator. It is a ternary operator, specialized with null values.???
is a classic comment in the code, when someone was surprised by some code.
Colon @ in PHP
:
is the label operator,label:
, that works with goto. It is also the other half of the ternary operator.::
is the scope resolution operator, which reachs data at the class level, and not at the object level.:::
is not supported.
Exclamation ! in PHP
!
is the negation operator: it turns any value into its boolean opposite.!!
is the negation negation operator. It is basically a shorter(boolean)
cast operator, and a barbarism coming from other languages.!!!
is the negation operator, but three times longer to write.
Arobase @ in PHP
@
is the noscream operator, which mutes error reporting on a syntax.@@
is the noscream operator, which mutes error reporting on a error-muted expression. It is in case the code should really not emit errors.@@@
is the noscream operator, which mutes error reporting on a doubly error-muted expression. It is in case the code should really really, and I mean really, not emit errors.
1-2-3 operators are fun!
It is interesting to discover the different meanings of an symbol as it is repeated. It might vary a lot in meaning and usage.
It is also interesting to realise that some of these repeated symbol operators are not used yet, and might be promoted to an actual PHP features. Replacing range(1, 19)
by 1..19
would be a nice new feature for PHP 8.5.
We could also dream of :::
, |||
, ___
or even quadruples operators ....
or @@@@
. Well, sometimes, we already have them!